Java中级测试题三-文件与流(4-4)
原创约 1338 字大约 4 分钟...
Java中级测试题三-文件与流(4-4)
注意
本博文仅供学术研究和交流参考,严禁将其用于商业用途。如因违规使用产生的任何法律问题,使用者需自行负责。
- 在本机的磁盘系统中,找一个文件夹,利用File类的提供方法,列出该文件夹中的所有文件的文件名和文件的路径,执行效果如下:
路径是xxx的文件夹内的文件有:
文件名:abc.txt
路径名:c:\temp\abc.txt
--------------------------------------------
文件名:def.txt
路径名:c:\temp\def.txt
package highchapter3;
import java.io.File;
public class ReadFile {
/*
* 1、在本机的磁盘系统中,找一个文件夹,利用File类的提供方法,
* 列出该文件夹中的所有文件的文件名和文件的路径,执行效果如下:
* 路径是xxx的文件夹内的文件有:
*
*/
static int totol = 0;
static int dirTotol = 0;
static String showFile(String path) {
File file = new File(path);
String [] fileArr = file.list();
for(int i=0;i<fileArr.length;i++) {
String nowPath = path+"\\"+fileArr[i];
File file1 = new File(nowPath);
if(file1.isDirectory()) {
showFile(nowPath);
dirTotol++;
}
else {
System.out.println("文件名:"+file1.getName());
System.out.println("路径名:"+nowPath);
totol++;
System.out.println("-------------------------------------");
}
}
return "";
}
public static void main(String[] args) {
System.out.println("列出该文件夹中的所有文件的文件名和文件的路径");
showFile("D:\\\\Sublime");
System.out.println("总共有"+totol+"个文件,文件夹有"+dirTotol+"个");
}
}
- 编写一个java程序实现文件复制功能,要求将d:/io/copysrc.doc中的内容复制到d:/io/copydes.doc中。
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
public class CopyFile {
public static void cyfile() {
File source = new File("d:/io/copysrc.doc");
//判断是否有源文件。如果没有则创建一个
if(!source.exists()) {
try {
source.createNewFile();
} catch (IOException e) {
e.printStackTrace();
}
}
//目标文件不用判断会自动创建
File dest = new File("d:/io/copydes.doc");
try(
BufferedReader br = new BufferedReader(new FileReader(source));
BufferedWriter bw = new BufferedWriter(new FileWriter(dest));
) {
String content = null;
while((content = br.readLine()) != null) {
bw.write(content);
bw.newLine();
}
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
cyfile();
}
}
- 创建c:/test.txt文件并在其中输入"hello world"创建一个输入流读取该文件中的文本并且把小写的l变成大写L再利用输出流写入到d:\test.txt中
3.1 实现步骤:
3.1.1 在本地硬盘C盘下创建一个文件test.txt
3.1.2 创建一个包含main()方法的类,并在main中编写代码
3.1.3 运行代码并且测试结果
3.2 实现过滤器的功能
效果显示:
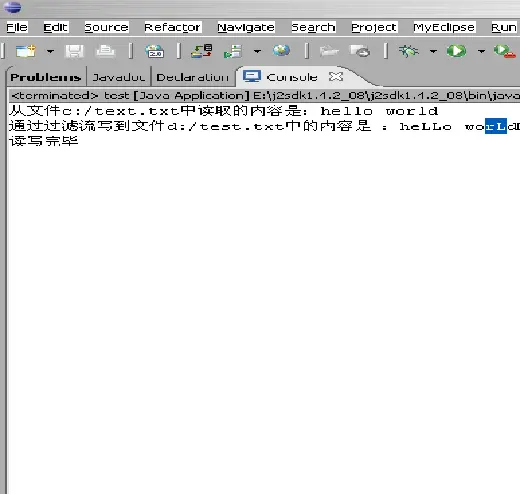
package highchapter3;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
public class Topic3 {
/*
* 3、创建c:/test.txt文件并在其中输入"hello world"
* 创建一个输入流读取该文件中的文本
* 并且把小写的l变成大写L再利用输出流写入到d:\test.txt中 [必做题]
* 3.1 实现步骤:
* 3.1.1 在本地硬盘C盘下创建一个文件test.txt 19:15
* 3.1.2 创建一个包含main()方法的类,并在main中编写代码 19:16
* 3.1.3 运行代码并且测试结果
* 3.2 实现过滤器的功能 效果显示:
*/
public static void crefile() {
File file = new File("file","test.txt");
if(!file.exists()) {
try {
file.createNewFile();
} catch (IOException e) {
e.printStackTrace();
}
}
//我是直接用代码输入文本
FileOutputStream fos = null;
String content = "hello world";
byte[] b = content.getBytes();
try {
fos = new FileOutputStream(file);
for(int i = 0;i < b.length;i++) {
try {
fos.write(b[i]);
} catch (IOException e) {
e.printStackTrace();
}
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}finally {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}//创建并 插入内容结束
//此处我将不按照题目要求路径放咯。。点解?因为我懒。。。。。。
File dest = new File("file","test2.txt");
try (
BufferedReader br = new BufferedReader(new FileReader(file));
BufferedWriter bw = new BufferedWriter(new FileWriter(dest));
){
String newContent = br.readLine().replace("l","L");
bw.write(newContent);
System.out.println("从文件file/test.txt中读取的内容是:" + br.readLine());
System.out.println("通过过滤写到 文件file/test2.txt中的内容是:" + newContent);
} catch (IOException e1) {
e1.printStackTrace();
}
}
public static void main(String[] args) {
crefile();
}
}
效果如下:
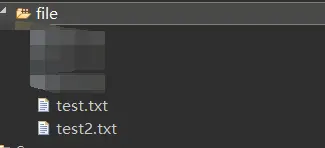
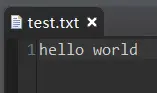
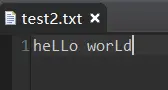
- 在程序中创建一个Student类型的对象,并把对象信息保存到d:/io/student.txt文件中,然后再从文件中把Student对象的信息读出显示在控制台上,Student类的描述如下:
package higchapter3;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.Serializable;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class Student implements Serializable{
/**
*
*/
private static final long serialVersionUID = 1L;
/*
* 4、在程序中创建一个Student类型的对象,并把对象信息保存到d:/io/student.txt文件中,
* 然后再从文件中把Student对象的信息读出显示在控制台上,Student类的描述如下:
*
*/
private Integer id;
private String name;
private Date birth;
public Student(Integer id, String name, Date birth) {
super();
this.id = id;
this.name = name;
this.birth = birth;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Date getBirth() {
return birth;
}
public void setBirth(Date birth) {
this.birth = birth;
}
@Override
public String toString() {
return "Student [id=" + id + ", name=" + name + ", birth=" + birth + "]";
}
//测试类
public static void main(String[] args) {
String birth = "1999-09-12";
SimpleDateFormat sf=new SimpleDateFormat("yyyy-MM-dd");
Date dateBirth = null;
try {
dateBirth=sf.parse(birth);
} catch (ParseException e1) {
e1.printStackTrace();
}
Student stu = new Student(100,"suwukong",dateBirth);
File file = new File("file","stu.txt");
if(!file.exists()) {
try {
file.createNewFile();
} catch (IOException e) {
e.printStackTrace();
}
}
File readFile = new File("file","stu.txt");
try (
ObjectOutputStream os = new
ObjectOutputStream(new FileOutputStream(file));
ObjectInput is = new ObjectInputStream
(new FileInputStream(readFile));
)
{
os.writeObject(stu);//写入
Student stu2 = (Student) is.readObject();//读取
System.out.println(stu);
} catch (Exception e) {
e.printStackTrace();
}
}
}
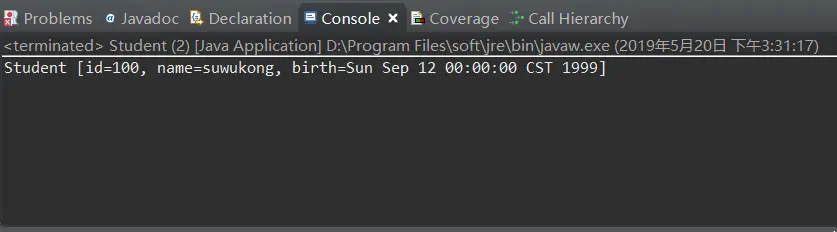
分割线
相关信息
以上就是我关于 Java中级测试题三-文件与流(4-4) 知识点的整理与总结的全部内容,希望对你有帮助。。。。。。。
Powered by Waline v2.15.4