JavaGUI界面编程-安装WindowBuilder插件并新建JFrame简单窗体 页面交互 常用的表单域 获取表单域的值
原创约 1258 字大约 4 分钟...
获取表单域的值.md
252-JavaGUI界面编程-安装WindowBuilder插件并新建JFrame简单窗体&页面交互 & 常用的表单域&注意
本博文仅供学术研究和交流参考,严禁将其用于商业用途。如因违规使用产生的任何法律问题,使用者需自行负责。
概念
在 Eclipse的 dropins 文件夹 放入 WinBuilder_v1.8.0_mars 文件
重启 Eclipse 若 window--> preferences --> 下有WindowBuilder 则安装成功
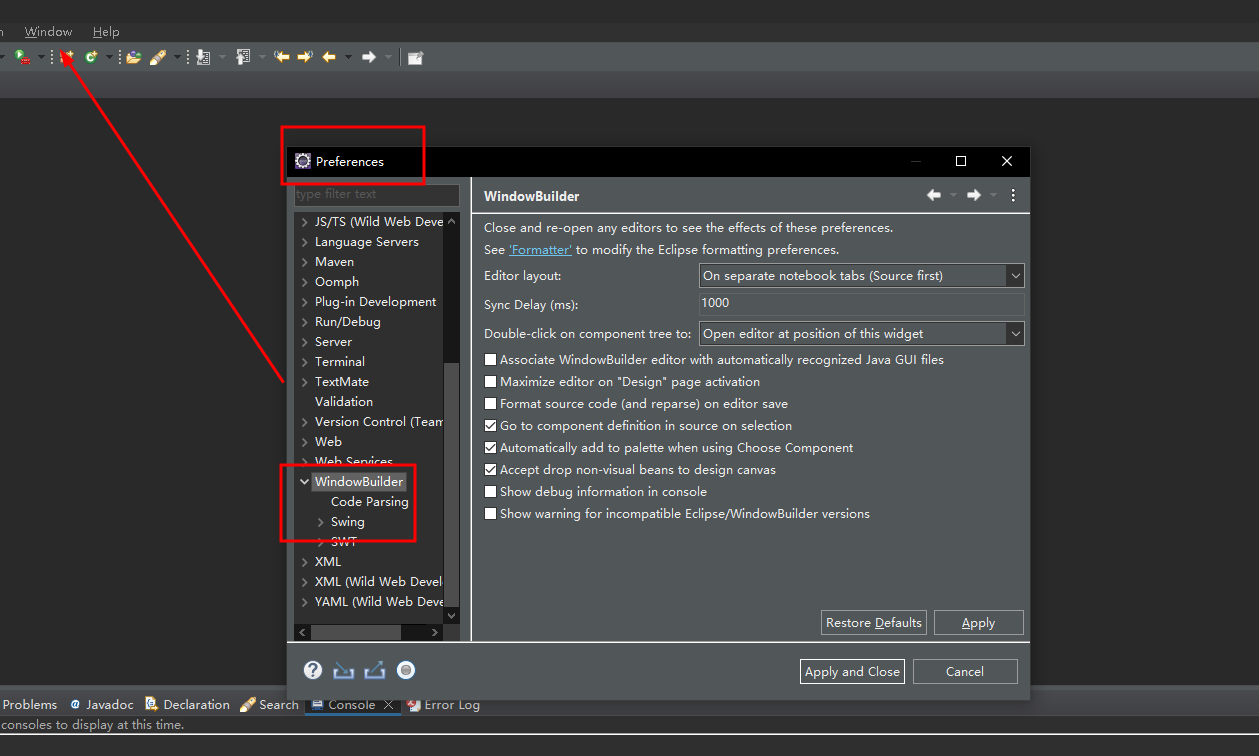
示例代码
package com.tencent.chapter06.gui;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.ButtonGroup;
import javax.swing.DefaultComboBoxModel;
import javax.swing.JButton;
import javax.swing.JCheckBox;
import javax.swing.JComboBox;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JRadioButton;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.JTextField;
public class AddEmpPanel extends JPanel {
private JTextField txt_empno;
private JTextField txt_ename;
private JPasswordField txt_password;
private final ButtonGroup buttonGroup = new ButtonGroup();
private JRadioButton rdb_man;
private JRadioButton rdb_female;
private JCheckBox cbx_dajidali;
private JCheckBox cbx_code;
private JCheckBox cbx_basketball;
private JCheckBox cbx_sing;
private JTextArea txt_demo;
private JComboBox combx_address;
/**
* Create the panel.
*/
public AddEmpPanel() {
setLayout(null);
JLabel label = new JLabel("\u5458\u5DE5\u7F16\u53F7\uFF1A");
label.setBounds(46, 43, 72, 15);
add(label);
txt_empno = new JTextField();
txt_empno.setText("123");
txt_empno.setBounds(128, 35, 110, 31);
add(txt_empno);
txt_empno.setColumns(10);
JLabel label_1 = new JLabel("\u5458\u5DE5\u59D3\u540D\uFF1A");
label_1.setBounds(321, 43, 72, 15);
add(label_1);
txt_ename = new JTextField();
txt_ename.setBounds(403, 36, 126, 28);
add(txt_ename);
txt_ename.setColumns(10);
JLabel label_2 = new JLabel("\u5458\u5DE5\u5BC6\u7801\uFF1A");
label_2.setBounds(46, 88, 72, 15);
add(label_2);
txt_password = new JPasswordField();
txt_password.setBounds(128, 80, 110, 31);
add(txt_password);
JLabel label_3 = new JLabel("\u6027 \u522B\uFF1A");
label_3.setBounds(321, 88, 72, 15);
add(label_3);
rdb_man = new JRadioButton("\u7537");
buttonGroup.add(rdb_man);
rdb_man.setBounds(403, 84, 46, 23);
add(rdb_man);
rdb_female = new JRadioButton("\u5973");
buttonGroup.add(rdb_female);
rdb_female.setBounds(475, 84, 54, 23);
add(rdb_female);
JLabel label_4 = new JLabel("\u7231 \u597D\uFF1A");
label_4.setBounds(46, 142, 72, 15);
add(label_4);
cbx_dajidali = new JCheckBox("\u5927\u5409\u5927\u5229");
cbx_dajidali.setBounds(128, 138, 82, 23);
add(cbx_dajidali);
cbx_code = new JCheckBox("\u5199\u4EE3\u7801");
cbx_code.setBounds(214, 138, 72, 23);
add(cbx_code);
cbx_basketball = new JCheckBox("\u7BEE\u7403");
cbx_basketball.setBounds(288, 138, 54, 23);
add(cbx_basketball);
cbx_sing = new JCheckBox("\u5531\u6B4C");
cbx_sing.setBounds(350, 138, 72, 23);
add(cbx_sing);
JLabel label_5 = new JLabel("\u7C4D \u8D2F\uFF1A");
label_5.setBounds(46, 184, 72, 15);
add(label_5);
combx_address = new JComboBox();
combx_address.setModel(new DefaultComboBoxModel(new String[] {"", "\u5E7F\u5DDE\u5E02", "\u6C55\u5934\u5E02", "\u6F6E\u5DDE\u5E02", "\u6DF1\u5733\u5E02", "\u6E5B\u6C5F\u5E02", "\u6C5F\u95E8\u5E02"}));
combx_address.setBounds(128, 181, 89, 21);
add(combx_address);
JLabel label_6 = new JLabel("\u5907 \u6CE8\uFF1A");
label_6.setBounds(46, 242, 72, 15);
add(label_6);
JScrollPane scrollPane = new JScrollPane();
scrollPane.setBounds(124, 237, 230, 78);
add(scrollPane);
txt_demo = new JTextArea();
txt_demo.setLocation(128, 0);
scrollPane.setViewportView(txt_demo);
txt_demo.setLineWrap(true);
//新增
JButton button = new JButton("\u65B0\u589E");
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
//员工编号
String empno = txt_empno.getText();
//员工姓名
String ename = txt_ename.getText();
//密码
char[] passwordArray = txt_password.getPassword();
//性别
String sex = "不详";
if(rdb_man.isSelected())
{
sex = rdb_man.getText();
}
else if(rdb_female.isSelected())
{
sex = rdb_female.getText();
}
//爱好
String hobby = "";
if(cbx_dajidali.isSelected())
{
hobby += cbx_dajidali.getText() + ";";
}
if(cbx_code.isSelected())
{
hobby += cbx_code.getText() + ";";
}
if(cbx_basketball.isSelected())
{
hobby += cbx_basketball.getText() + ";";
}
if(cbx_sing.isSelected())
{
hobby += cbx_sing.getText() + ";";
}
//籍贯
String address = (String)combx_address.getSelectedItem();
//备注
String demo = txt_demo.getText();
System.out.println("员工编号:" + empno + "\n"
+ " 员工姓名:" + ename + "\n"
+ " 密码:" + new String(passwordArray) + "\n"
+ " 性别:" + sex + "\n"
+ " 爱好:" + hobby + "\n"
+ " 籍贯:" + address + "\n"
+ " 备注:" + demo + "\n");
}
});
button.setBounds(535, 319, 93, 23);
add(button);
JButton button_1 = new JButton("\u91CD\u7F6E");
button_1.setBounds(417, 319, 93, 23);
add(button_1);
}
}
package com.tencent.chapter06.gui;
import java.awt.EventQueue;
import java.awt.Toolkit;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JPanel;
import javax.swing.JSeparator;
import javax.swing.JToolBar;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
import javax.swing.border.EmptyBorder;
public class MainFrame extends JFrame {
private JPanel contentPane;
private JPanel panel;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
MainFrame frame = new MainFrame();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
static{
try {
// UIManager.setLookAndFeel("com.sun.java.swing.plaf.nimbus.NimbusLookAndFeel");//Nimbus风格,jdk6 update10版本以后的才会出现
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());//当前系统风格
// UIManager.setLookAndFeel("com.sun.java.swing.plaf.motif.MotifLookAndFeel");//Motif风格,是蓝黑
// UIManager.setLookAndFeel(UIManager.getCrossPlatformLookAndFeelClassName());//跨平台的Java风格
// UIManager.setLookAndFeel("com.sun.java.swing.plaf.windows.WindowsLookAndFeel");//windows风格
// UIManager.setLookAndFeel("javax.swing.plaf.windows.WindowsLookAndFeel");//windows风格
// UIManager.setLookAndFeel("javax.swing.plaf.metal.MetalLookAndFeel");//java风格
// UIManager.setLookAndFeel("com.apple.mrj.swing.MacLookAndFeel");//待考察,
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (InstantiationException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
} catch (UnsupportedLookAndFeelException e) {
e.printStackTrace();
}
}
/**
* Create the frame.
*/
public MainFrame() {
setIconImage(Toolkit.getDefaultToolkit().getImage("E:\\Tencent\\MySeniorProject\\icon\\layers.png"));
setTitle("\u96C7\u5458\u7BA1\u7406\u7CFB\u7EDF");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 761, 449);
JMenuBar menuBar = new JMenuBar();
setJMenuBar(menuBar);
JMenu menu = new JMenu("\u5458\u5DE5\u7BA1\u7406");
menuBar.add(menu);
JMenu menu_4 = new JMenu("\u65B0\u589E\u5458\u5DE5");
menu.add(menu_4);
JMenuItem menuItem = new JMenuItem("\u65B0\u589E\u666E\u901A\u5458\u5DE5");
menu_4.add(menuItem);
JMenuItem menuItem_1 = new JMenuItem("\u65B0\u589E\u7BA1\u7406\u5458");
menu_4.add(menuItem_1);
JMenuItem menuItem_2 = new JMenuItem("\u67E5\u8BE2\u5458\u5DE5");
menu.add(menuItem_2);
JSeparator separator = new JSeparator();
menu.add(separator);
JMenuItem menuItem_3 = new JMenuItem("\u9000\u51FA");
menu.add(menuItem_3);
JMenu menu_1 = new JMenu("\u90E8\u95E8\u7BA1\u7406");
menuBar.add(menu_1);
JMenu menu_2 = new JMenu("\u5C97\u4F4D\u7BA1\u7406");
menuBar.add(menu_2);
JMenu menu_3 = new JMenu("\u7CFB\u7EDF\u8BBE\u7F6E");
menuBar.add(menu_3);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(null);
JToolBar toolBar = new JToolBar();
toolBar.setBounds(0, 0, 745, 35);
contentPane.add(toolBar);
//新增员工
JButton button = new JButton("");
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
//1.先移除原panel中所有的内容
panel.removeAll();
panel.repaint();
//2.再想panel中添加新的内容(新增员工的界面)
AddEmpPanel addEmpPanel = new AddEmpPanel();
addEmpPanel.setBounds(0, 0, 745, 352);
panel.add(addEmpPanel);
}
});
button.setToolTipText("\u65B0\u589E\u5458\u5DE5");
button.setIcon(new ImageIcon("E:\\Tencent\\MySeniorProject\\icon\\add.png"));
toolBar.add(button);
JButton button_1 = new JButton("");
button_1.setToolTipText("\u8C03\u8F6C\u90E8\u95E8");
button_1.setIcon(new ImageIcon("E:\\Tencent\\MySeniorProject\\icon\\layout_edit.png"));
toolBar.add(button_1);
JButton button_2 = new JButton("");
button_2.setToolTipText("\u8C03\u6574\u85AA\u8D44");
button_2.setIcon(new ImageIcon("E:\\Tencent\\MySeniorProject\\icon\\money.png"));
toolBar.add(button_2);
panel = new JPanel();
panel.setBounds(0, 38, 745, 352);
contentPane.add(panel);
panel.setLayout(null);
JLabel lblNewLabel = new JLabel("");
lblNewLabel.setIcon(new ImageIcon("E:\\Tencent\\MySeniorProject\\icon\\bg.jpg"));
lblNewLabel.setBounds(0, 0, 745, 352);
panel.add(lblNewLabel);
//窗体居中
this.setLocationRelativeTo(null);
}
}
分割线
相关信息
以上就是我关于 JavaGUI界面编程-安装WindowBuilder插件并新建JFrame简单窗体&页面交互 & 常用的表单域&获取表单域的值 知识点的整理与总结的全部内容,希望对你有帮助。。。。。。。
Powered by Waline v2.15.4