Java多线程编程-常用方法-wait() notify()方法
原创约 633 字大约 2 分钟...
方法.md
247-Java多线程编程-常用方法-wait() notify()注意
本博文仅供学术研究和交流参考,严禁将其用于商业用途。如因违规使用产生的任何法律问题,使用者需自行负责。
概念
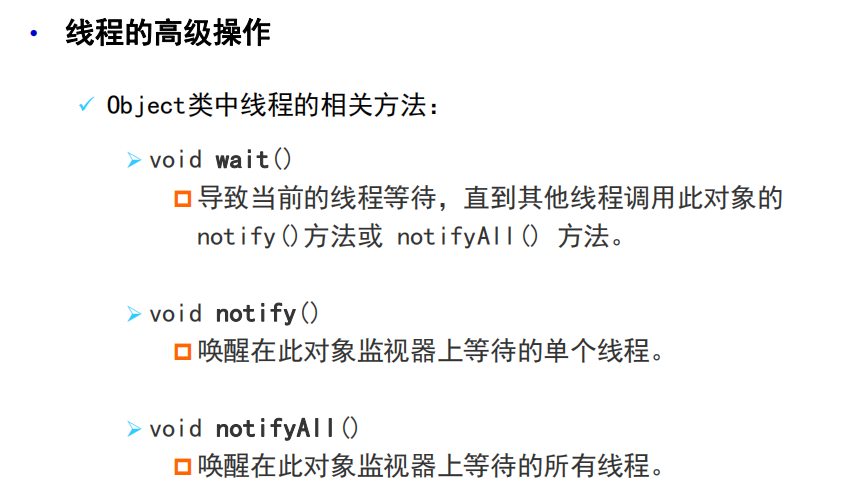
示例代码
package com.tencent.chapter04.生产消费;
//消费者
public class Customer extends Thread {
private Store store;
public Customer(Store store)
{
this.store = store;
}
@Override
public void run() {
while(true)
{
synchronized (store) {//如果当前线程不是此对象监视器的所有者,则抛出IllegalMonitorStateException
//当仓库的当前容量 == 0是,停止消费,等待生产者进行生产
while(store.count <= 0)//为了避免虚假唤醒,不要使用if判断,使用while循环判断
{
try {
store.wait();//导致当前的线程等待,直到其他线程调用此store对象的 notify()方法或 notifyAll() 方法
} catch (InterruptedException e) {
e.printStackTrace();
}
}
//出库
store.out();
//唤醒生产者进行生产
store.notify();
//休眠一秒
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
package com.tencent.chapter04.生产消费;
//生产者
public class Producer extends Thread {
private Store store;
public Producer(Store store)
{
this.store = store;
}
@Override
public void run() {
while(true)
{
synchronized (store) {//如果当前线程不是此对象监视器的所有者,则抛出IllegalMonitorStateException
//当当前容量 == 最大容量,停止生产,等待消费者消费
while(store.count >= store.MAX_COUNT)//为了避免虚假唤醒,不要使用if判断,使用while循环判断
{
try {
store.wait();//导致当前的线程等待,直到其他线程调用此store对象的 notify()方法或 notifyAll() 方法
} catch (InterruptedException e) {
e.printStackTrace();
}
}
//入库
store.in();
//唤醒消费者进行消费
store.notify();
//休眠一秒
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
package com.tencent.chapter04.生产消费;
//仓库
public class Store {
//最大容量
final int MAX_COUNT = 5;
//当前容量
int count;
//入库
public void in()
{
System.out.println("入库前库存:" + count);
count++;
System.out.println("入库后库存:" + count);
}
//出库
public void out()
{
System.out.println("出库前库存:" + count);
count--;
System.out.println("出库后库存:" + count);
}
}
package com.tencent.chapter04.生产消费;
public class Test {
public static void main(String[] args) {
//创建一个仓库
Store store = new Store();
//生产者
Producer producer = new Producer(store);
producer.start();
//消费者
Customer customer = new Customer(store);
customer.start();
Customer customer2 = new Customer(store);
customer2.start();
}
}
分割线
相关信息
以上就是我关于 Java多线程编程-常用方法-wait() notify()方法 知识点的整理与总结的全部内容,希望对你有帮助。。。。。。。
Powered by Waline v2.15.4