JavaScript实践数据结构和算法——希尔排序
原创约 351 字大约 1 分钟...
JavaScript实践数据结构和算法——希尔排序
注意
本博文仅供学术研究和交流参考,严禁将其用于商业用途。如因违规使用产生的任何法律问题,使用者需自行负责。
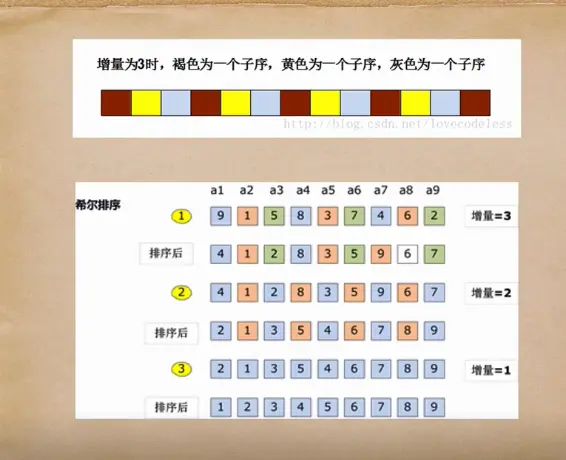
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>希尔排序</title>
</head>
<body>
<script>
var CArray = function () {
this.dataStore = [10,8,3,2,5,9,4,7,35,47,20];
this.shellsort = shellsort;
this.gaps = [5,3,1];
this.swap = swap;
this.dynamiSort = dynamiSort;
};
function shellsort(arr,index1,index2) {
for(var g = 0;g<this.gaps.length;g++){
for(var i = this.gaps[g];i<this.dataStore.length;i++){
var temp = this.dataStore[i];
for(var j=i;j>=this.gaps[g]&&this.dataStore[j-this.gaps[g]]>temp;j-=this.gaps[g]){
this.dataStore[j] = this.dataStore[j-this.gaps[g]];
}
this.dataStore[j] = temp;
}
console.log("调换后",this.dataStore);
}
}
//交换数组
function swap(arr,index1,index2) {
var temp = arr[index1];
arr[index1] = arr[index2];
arr[index2] = temp;
}
function dynamiSort() {
var N = this.dataStore.length;
var h = 1;
while (h<N/3){
h =3*h+1;
}
while (h>=1){
for(var i = h;i<N;i++){
for(var j = i;j>=h && this.dataStore[j]<this.dataStore[j-h];j=j-h){
this.swap(this.dataStore,j,j-h);
}
}
h = (h-1)/3;
}
}
var mynums = new CArray();
// mynums.shellsort();
mynums.dynamiSort();
console.info(mynums.dataStore);
</script>
</body>
</html>
- 得到结果:
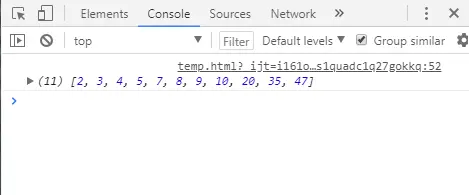
分割线
相关信息
以上就是我关于 JavaScript实践数据结构和算法——希尔排序 知识点的整理与总结的全部内容,希望对你有帮助。。。。。。。
Powered by Waline v2.15.4